March 15th, 2010
When starting your first Aspnet MVC2 project you might immediately run into compilation errors like these:
Error 1 The type or namespace name ‘Controllers’ does not exist in the namespace ‘MvcApplication1’ (are you missing an assembly reference?) C:\myprojectpath\MvcApplication1.Tests\Controllers\HomeControllerTest.cs 8 23 MvcApplication1.TestsError 3 The type or namespace name ‘Models’ does not exist in the namespace ‘MvcApplication1’ (are you missing an assembly reference?) C:\myprojectpath\MvcApplication1.Tests\Controllers\AccountControllerTest.cs 10 23 MvcApplication1.Tests
Error 4 The type or namespace name ‘AccountController’ could not be found (are you missing a using directive or an assembly reference?) C:\myprojectpath\MvcApplication1.Tests\Controllers\AccountControllerTest.cs 317 24 MvcApplication1.Tests
The origin of the problem is that the test project doesn’t reference the web project. Just add the reference and you’re good to go.
I haven’t found the solution on google yet so I write this here to someones possible future aid.
March 4th, 2010
>Due to some reason unknown to man the team behind VBNet decided that the developer should not be able to see if someone listened to your event or not. It is good in most cases but very bad in another.
In Csharp raising an event goes something like this:
var ev = MyEvent;
if( null != ev ){ ev( this, args ); }
Just look through the snippets (look in the context menu in the editor) and you will find the correct way to raise an event in Csharp.
VBNet on the other hand hides the if statement and the temporary variable from the developer. It is enough to write
RaiseEvent Me, args
and the event will be raise if there is someone listening – no need to check for null.
But what if you want to check if someone is listening. In my case I needed to know if the custom control was properly wired into the scaffolding of the application; the user control sometimes whished for more information and I had to know if someone was listening.
In Csharp this would have been easy with a check for null. But VBNet…
The solution is hidden. Just check the variable “MyEventEvent“. This means that your MyClick event has a hidden variable MyClickEvent that you can check for like this:
If MyClickEvent IsNot Nothing Then
I don’t like to use hidden and unoffical solutions but this is the only way I know of.
Honor those who should.
March 4th, 2010
>I just started another blog which only presents ideas yet to be implemented.
http://selfelected.wordpress.com/
Feel free to implement anyone of them.
February 4th, 2010
The text and code below is only tested for Winform. WPF might behave differently; hopefully behaves differently.
I won’t go into any details on why the ISite.DesignMode is good and sometimes even crucial to have. It is much better done in the link at the bottom.
First out there is unfortunately a caveat with DesignMode, it doesn’t work with inherited forms and user controls. This is a problem since I always inherit from the base form in my projects and tell others to do the same. It also doesn’t work inside the form’s or the user control’s constructor and then by any method that is called by the constructor. Long call chains to might make this hard to track down.
Secondly there is another way to check for design mode and that is to check for the LicenseManager.UsageMode but this in turn doesn’t work in event handlers.
Thirdly one can set a flag in the base Form and base UserControl. But this doesn’t solve the problem since we cannot be sure with what to set this value.
Fourthly is a workaround where one can check for the name of the process itself which is Visual Studio’s process name (devenv) in case of design mode. This process name might change in future releases of Visual Studio and is also different for other IDEs.
Fifthly is other process information like the name of the module and stuff. Check into the Process and you’ll see that the app is called MyApp.vshost.exe while being debugged.
The fourth solution (processname=devenv) seems to be the most viable but I believe there is something useful in the fifth (other process information). There must be a way to notice that the name of all your code doesn’t match the name of the running environment; namespace, assembly name, whatever. I still haven’t figured out how though.
Below is some code to copy-paste.
It is not 100% correct though since the DesignMode property isn’t virtual. This might end with some really tough-to-track-down bugs where one iterates the forms but don’t get the overloaded DesignMode flag. The code also doesn’t promise to work forever since there is a magic string naming the name of the Visual Studio process.
public partial class MyAppForm : System.Windows.Forms.Form{protected new bool DesignMode{get{return HelperMethods.IsDesignMode(this);}}}
public partial class MyAppUserControl : UserControl{protected new bool DesignMode{get{return HelperMethods.IsDesignMode(this);}}}
class HelperMethods{public static bool IsDesignMode(System.ComponentModel.Component x){var site = x as System.ComponentModel.ISite;return( null == site ? false : site.DesignMode ) ||System.ComponentModel.LicenseManager.UsageMode == System.ComponentModel.LicenseUsageMode.Designtime ||System.Diagnostics.Process.GetCurrentProcess().ProcessName == "devenv";}}
The code above is also in pastebin for easier copy-paste.
A good article is here: http://dotnetfacts.blogspot.com/2009/01/identifying-run-time-and-design-mode.html
There is a description of how to debug this here: http://brennan.offwhite.net/blog/2006/08/30/design-time-debugging-aspnet-20-in-visual-studio-2005/
Error 4 The type or namespace name ‘AccountController’ could not be found (are you missing a using directive or an assembly reference?) C:\myprojectpath\MvcApplication1.Tests\Controllers\AccountControllerTest.cs 317 24 MvcApplication1.Tests
January 28th, 2010
>
I didn’t think it was possible but it has been for years; to share a file between projects in a solution.
Add a file the normal way but instead of pressing Open, use the little dropdown arrow next to. Then you have the possibility to “link” instead of “add”.
This makes it possible to have one and only one file for many places. It can be a source code file or a readme or anything really.
I have yet to try it with TFS and SVN to make sure it doesn’t mess upp the version management.
Honor those who should.
On a side note: Sourcesafe has linking ability within itself. I miss it in TFS. Though: It is easy to lose the master file and keep copies wich makes strange icons. It also doesn’t play well with the VB6 editor when a file will be checked out and another not, but they should be, but the VB6 ide doesn’t understand it and you have to do an explicit checkout and… never mind. It was a long time ago.
January 24th, 2010
In Windows 7 (and possibly Vista) the keyboard layout is sometimes changed back to some sort of default.
The problem shows itself like this – I use the 1337 keyboard layout in Visual Studio. Then without warning the keyboard layout changes to US layout; a layout I don’t even have installed. The 1337 layout is based on US though so somehow it shines through.
To get back to 1337 I choose “Open the language bar” in the systray context menu. The language bar then pops up in another place (top of screen for me) and I can then choose keyboard layout.
The problem will reappear more times until I reboot.
January 16th, 2010
Way too many times have I written
void MyFunction( int aNumber){ MyHomemadeLogClass.Logg( "MyFunction", "aNumber=" + aNumber.ToString()); ...}
to get logging functionality.
It would be so nice to just write
void MyFunction( int aNumber){ MyHomemadeLogClass.Logg(); ...}
It is no hard to do. Use reflection to get the call stack to get to the method and the parameters. Convert all this to a readable string.
Unfortunately it is not that easy to log the contents of the parameters, one has to get into some sort of debugger area to do that.
class ReflectionUtility { private static System.Reflection.MethodBase GetCallingMethod() { return new System.Diagnostics.StackTrace().GetFrame(2).GetMethod(); } public static string GetCallingMethodFullNameReturnParametertypes() { return MethodFullNameReturnParametertypes(GetCallingMethod()); } private static string MethodFullNameReturnParametertypes(System.Reflection.MethodBase method) { return string.Format("{0} {1}.{2} ({3})", ((System.Reflection.MethodInfo)method).ReturnType, // System.Void, System.Int32 etc. method.DeclaringType.FullName, // MyNamespace.MyClass. method.Name, // MyMethod. string.Join(",", method.GetParameters().Select(p => p.ParameterType.ToString() + " " + p.Name).ToArray()) // () or (int) or (int,string) etc. ); } }
To use this in a Logging class one must implement a GetCallingCallingMethod or even worse, but it would make this example hard to read.
One can use the Conditional attribute to avoid the call when not debugging.
I also put the code on pastebin to make the copy-paste easier. (I have yet to find a good way to present code.)
Update: go to http://compulsorycat.googlecode.com for the source code in a LGPLd project.
January 9th, 2010
>
This is my visual setup of Visual Studio.
Notice how the real estate is used for tools I use and not for buttons that just happens to be there by default.
shift-alt-return maximises the window so not even the caption bar is visible. I know which program I am working in so I don’t need to waste the whole top for this.
I have removed all toolbars. The buttons I need I have put to the right of the menu.
There are also two macros in the menu bar, one for connecting the bugger to nunit and ditto to IIS.
I have set all toolbars to auto hide. When I debug, the call stack and autos (unfortunately hard to find among the menus with C# projects – open a VBNet project and see what it looks like), toolbox are visible.
I have also moved all toolbars to the right. When one is working heavily with the forms designer it is good to have the Toolbox toolbar constantly open on the left but only then, and for the 2% of the project I handle the forms I can move it there manually. The rest of the time I hack code and need nothing but code and debug tools.
I know shortcuts to almost all toolbars. Those I don’t know I don’t use that often anyway. I don’t know why some banjo player at Microsoft decided that underlines and shortcut tips should be invisible as default. Fix it through Tools->Customize.
This way I use all of the precious display area for stuff I have use of.
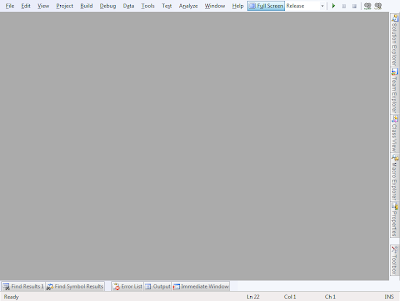
January 6th, 2010
When you want to populate a dropdown listbox with customers
class Customer{ int Id; string Name; ...}
and have to write
myDropdownListbox.DisplayMember = "Name";myDropdownListbox.DataMember = "Id";
or
myGridColumnCustomerName.DatatMeber = "Name";
you really get a sour taste in your mouth.
Having magic strings like above spread in your code is really bad since it always compiles but might fail runtime. This means more manual tests. Which in turns means a certain friction against renaming properties while refactoring.
I have been in way too many projects where one table has a field ItemNumber when it should have been ItemCount and where some fields are called Nr while another Number and these names are spread up in the layers to reach the presentation layer where they surface as magic strings like the examples above.
Luckily there is a solution in Dotnet 3 with LINQ. It isn’t the prettiest but it fails compile time when it should and that is considered a good thing.
( I won’t bother with explanation – just read the code. )
When you want to populate a dropdown listbox with customers
class Customer{ int Id; string Name; ...}
and have to write
myDropdownListbox.DisplayMember = "Name";myDropdownListbox.DataMember = "Id";
or
myGridColumnCustomerName.DatatMeber = "Name";
you really get a sour taste in your mouth.
Having magic strings like above spread in your code is really bad since it always compiles but might fail runtime. This means more manual tests. Which in turns means a certain friction against renaming properties while refactoring.
I have been in way too many projects where one table has a field ItemNumber when it should have been ItemCount and where some fields are called Nr while another Number and these names are spread up in the layers to reach the presentation layer where they surface as magic strings like the examples above.
Luckily there is a solution in Dotnet 3 with LINQ. It isn’t the prettiest but it fails compile time when it should and that is considered a good thing.
( I won’t bother with explanation – just read the code. )
// The Code.
<
class ReflectionUtility
{
public static string GetPropertyName<T, TReturn>(Expression<Func<T, TReturn>> expression)
{
MemberExpression body = (MemberExpression)expression.Body;
return body.Member.Name;
}
public static string GetMethodName<T, TReturn>(Expression<Func<T, TReturn>> expression)
{
var body = expression.Body as UnaryExpression;
var operand = body.Operand as MethodCallExpression;
var argument = operand.Arguments[2] as ConstantExpression;
var methodInfo = argument.Value as System.Reflection.MethodInfo;
return methodInfo.Name;
}
}
class MyClass
{
public int MyField;
public int MyPublicProperty { get; set; }
public string MyReadonlyProperty { get { return string.Empty; } }
public int MyMethod() { return 0; }
private MyClass() { } // To make sure the class doesn't need a default constructor.
}
class Program
{
static void Main(string[] args)
{
string fieldName = ReflectionUtility.GetPropertyName((MyClass x) => x.MyField);
Console.WriteLine(string.Format("MyClass.MyField:{0}", fieldName));
Debug.Assert("MyField" == fieldName);
string propertyName = ReflectionUtility.GetPropertyName((MyClass x) => x.MyPublicProperty);
Console.WriteLine(string.Format("MyClass.MyPublicProperty:{0}", propertyName));
Debug.Assert("MyPublicProperty" == propertyName);
propertyName = ReflectionUtility.GetPropertyName((MyClass x) => x.MyReadonlyProperty);
Console.WriteLine(string.Format("MyClass.MyReadonlyProperty :{0}", propertyName));
Debug.Assert("MyReadonlyProperty" == propertyName);
string methodName = ReflectionUtility.GetMethodName<MyClass, Func<int>>((MyClass x) => x.MyMethod);
Console.Write(string.Format("MyClass.MyMethod:{0}", methodName));
Debug.Assert("MyMethod" == methodName);
Console.Write(Environment.NewLine + "Press any key.");
Console.ReadKey();
}
< }
Honor those who should. (This link contains a comment regarding generic methods that I’d like to impolement in CompulsoryCat.)
Update: When copy-pasting through manoli.net some angle brackets got dropped. This is, hopefully, fixed now. Otherwise – get the source here: http://selfelected.pastebin.com/f77563a02
Update:
Check out Compulsorycat. It is my F/OSS library with some of these functions.
Honor those who should. (This link contains a comment regarding generic methods that I’d like to impolement in CompulsoryCat.)
Update: When copy-pasting through manoli.net some angle brackets got dropped. This is, hopefully, fixed now. Otherwise – get the source here: http://selfelected.pastebin.com/f77563a02
Update:
Check out Compulsorycat. It is my F/OSS library with some of these functions.
December 29th, 2009
A keyboard layout aimed at programmers for Windows and OSX.
It swaps numbers and characters in the top row so one doesn’t have to press shift for every parenthesis.
TL;DR
Most programming languages I have tried work better with a US keyboard layout than a Swedish. For instance my current language, C#, is riddled with {s and [s and these are a pain to use. One has to press the right Alt-button (labeled alt-gr) and then 7 or 8 respectively. Try this, it really twists your hand.
The US keyboard has {, [, ], } to the right of P and it works much better than the Swedish keyboard.
But then we have (, ), =, & and the rest above the number keys. Most languages use plenty of parenthesises and even though you don’t have to twist your hand it is still awkward to use two keys (and hands with a “proper” finger setting) to write these common characters. The French keyboard has solved this by flipping the number keys/shift setting. Press the key to get an ! and shift-key to get 1. If one write lots of number there is always the proper number keyboard to the right.
So the French keyboard is better for some other characters.
Then we have the Swedish characters å,ä and ö. Even though I usually hack in English I write text, outputs and user information in Swedish so these cannot be left out.
Thinking that there was no reason to have a second rate keyboard layout I fired up MSKLC from Microsoft and hacked together the 1337 keyboard layout. I have used it for a couple of years now in WinXP, Vista and Win7.
For almost all use other than programming I keep the Swedish layout so I have set leftshift-leftalt for a fast keyboard layout toggle.
Lastly: I do use touch typing and I recommend learning this for all serious software developers. I also have noticed that I have very little problem with switching keyboard layout, the fingers need a second to adjust and are then hitting the right key.
(For problems 1337 reverting to US see here.)