Archive for the ‘Code and Development’ Category
January 25th, 2013
If you are running your development on HTTP but testing, QA and production on HTTPS you might have stumbled on
Do you want to view only the web page content that was delivered securely?
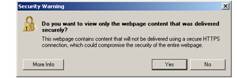
“Do you want to view only the webpage content that was delivered securely” dialogue.
The culprit might be your site loading stuff through HTTP while the site is run through HTTPS; the browser doesn’t like that.
Solution:
Instead of rewriting everything to HTTPS or create a deploy transformation to have different versions in dev, test and the rest just abandon the protocol.
E.g. use:
1
| <script type="text/javascript" src="//www.otherexamplesite.com/cdn/jquery.js"></script> |
instead of
1
| <script type="text/javascript" src="https://www.otherexamplesite.com/cdn/jquery.js"></script> |
Happy hacking!
January 13th, 2013
TL;DR
- You have to have knowledge of your types, either through tools or through syntax.
- Have different naming conventions for different languages.
- A strongly typed compiled language can have any naming convention but a weekly typed should have the types integrated in the variable names.
LONGER
Should one use Hungarian notation (sName, oCustomer), type suffixing (nameString, customerObject) or leave out the type (name, customer). The Rulez have come full circle and we are now back at keeping it simple so name and customer is what we write in coding standards nowadays. I am a die hard fan of compiled and strongly typed languages and I really don’t care. My compiler, my IDE and my plugin take care of finding misspellings and type mismatches.
But…
In my head I couldn’t get the simplest, and as I see it most modern naming convention (name, customer), to work together with the weekly typed javascript language. Keeping track of types in javascript is a problem and if one doesn’t get any help from the variable names – how does one do?
So I set out on a 5 day experiment.
I wrote some real production code with Aspnet Webforms, Knockout, Underscore, Jquery, Ajax and Web services returning Json.
I chose to name variables and methods in their simplest form.
1
| updateCustomers( customers ) |
which iterates and calls
1
| updateCustomer( customer ) |
and returns a bool if everything worked as expected.
My conclusion is that this is a bad idea.
I spent way too much time debugging and tracing the-object-property-having an-array-of-another-object-type and whether the final property had a plural “s” or not. The example is not contrived; I had a view model object containing an object which contained a list of Customers which contained properties, or a property which was a list.
To add insult to injury – Knockout was involved so I had to trace through method calls which made the debugging even more tedious. (don’t get me wrong – Knockout still does it’s work properly – it is my own objects and their relations and above all naming that trips me)
After this real world experiment I now have a case for having the type in the identifier names.
1
| bUpdateCustomers( aCustomer ) |
which iterates the the parameter and calls
1
| bUpdateCustomer( oCustomer ) |
I am not saying hungarian notation is better than suffixing the type but it makes for shorter names. Which makes for less possibility to mistype.
[ before gong berserk on Hungarian notation – please check into the difference between System and Apps. ]
December 17th, 2012
With VS2010; Blend was shipped as a stand alone product. With VS2012; Blend was bundled. Almost.
The Blend that comes with VS2012 only does Win8 ModernUI apps.
But there is a remedy: download Blend preview from here: http://bit.ly/U3Opxh. Login might be required.
November 28th, 2012
Every so often (but more seldom) I hear that IE doesn’t have a debugger.
“What?” I say. “I have been using a debugger in IE for many years.”
It is (was?) hard to find though. One way was to install Visual studio. Another to install MSOffice. The third was (is) to follow this forgotten, seldom mentioned but still working link to download. I have recently used it for debugging an unpatched IE7 on WinXP.
Today the debugger is one F12 press away.
<rant>The IE debugger has shortcuts which is a must for debugging. Mousing is doable but not for serious work. (YMMV) Chrome, Opera and Firefox have shortcuts too but they mix with the debugee – try setting the focus on the debugee form and then press a shortcut; nothing happens. By the time you, dear reader, read this the problem might have been solved but for now I am right. <mad-professor-laughter/></rant>
November 22nd, 2012
For my fellow googlewithbingers out there:
If you run a setup like mine with Win8 running on OSX through Parallels and your VS2012 sometimes stops responding to key presses; activate another application, then press the windows/command button for getting the start screen and then choose VS2012. Your already running VS gets focus and the keyboard is restored.
Don’t know why this works. There are certainly better workarounds. The problem with probably go away in the future. But for now it saves me.
November 14th, 2012
I am not doing yet a write up on how to write classes in javascript; instead I just link to the one stop shop by Douglas Crockford. It contains examples and explanations.
By the way – if you are serious about Javascript; do read his book.
November 1st, 2012
The Search programs and files command doesn’t work as good in Windows 8 as Windows 7 or Vista. In fact, it only does a fraction of its predecessor.
As a developer I often manipulate windows services and hence use the Services app in Control panel. In Win7 I just press the windows button and write services.
One can find Services in Windows 8 too but it doesn’t come as easy. One has to start searching (just press a letter at the start screen) but then choose to search in Settings through pressing arrow-down arrow-down enter.
I have a workaround to find Services and it is to go through the normal Control panel->Administration path and copy the Services shortcut. I then pin this shortcut to Windows8 start screen.
Next time I want to open the Services I just write services and hey presto – it shows up in the search result.
One can do this for the usual programs one wants to get to.
It took the original start button over 10 years to develop into what it is today. I don’t blame Microsoft for not getting it right at first try and I look forward to future refinements.
October 22nd, 2012
If you have a WCF solution it is quite easy to log the calls. (probably not always but it worked for me)
I used http://stackoverflow.com/questions/9232819/wcf-tracing-seems-to-be-truncating and http://msdn.microsoft.com/en-us/library/ms730064.aspx as info.
October 4th, 2012
I recently released some Dotnet open source functionality for doing safer string formatting.
Think like this:
When formatting strings in Dotnet C#/Vbnet string.Format is handy. But it always carries the risk of failing runtime when the format string is incorrect.
When you are doing (error) logging with strings (in a file) you want the logging to proceed without failing. Ever.
SafeFormat(…) or it’s extension variant “…”.SFormat(…) does just this. It formats exactly as string.Format does (it uses string.Format internally) but in case of a wrongly formatted format string it doesn’t throw an exception but instead does as good as it can and outputs the data it can gather together with a comment that something is broken.
Get all the shiny here: https://github.com/LosManos/CompulsoryCow
September 27th, 2012
Jag höll 2 presentationer på denna höstens OpKoKo, bägge 10-minuters blixtintro. En var om Netduino och en om grafdatabaser i allmänhet och Neo4j i synnerhet.
Till Netduino-föreläsningen finns en presentation (det som ofta kallas “en powerpoint”) men till Neo4j inte.